Why I Love Go
2024-09-14 Back to posts
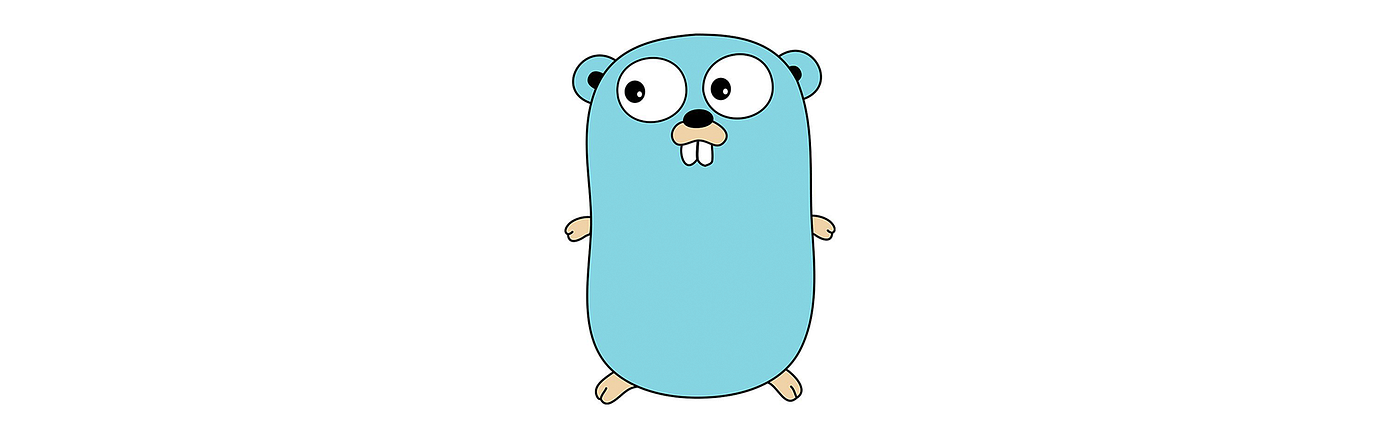
Why I Love Go
Introduction
As someone who enjoys writing command-line tools and working on backend services, Go has become one of my favorite languages for many reasons. It strikes a perfect balance between simplicity and performance, making it ideal for building scalable and high-performance applications.
Go was created by Google engineers with a focus on simplicity, concurrency, and speed, which makes it stand out for anyone developing modern software.
In this post, I’ll highlight what makes Go so great and share a code example that showcases one of its key features.
Simplicity and Readability
One of the main reasons I love Go is its minimalistic syntax.
Unlike other languages that tend to become bloated with features, Go keeps things simple.
You don’t need to dive into complex design patterns or unnecessary abstractions, making code easy to read and maintain. This makes it perfect for writing tools that are meant to “just work” without unnecessary complexity.
Go’s focus on clarity also reduces the learning curve for new developers.
Performance Without Sacrificing Developer Productivity
Go compiles down to native machine code, so the performance is close to C or C++, but without the memory management headaches.
This is especially important when building apps that require fast execution, such as API services or real-time applications.
At the same time, Go has a garbage collector, meaning developers don’t have to manually manage memory—providing a great balance between control and ease of use.
Concurrency Model
Concurrency is where Go truly shines. Its built-in support for goroutines allows you to easily run multiple tasks concurrently without dealing with threads or complex locking mechanisms. The concurrency model is simple but powerful, making it perfect for writing network servers, parallel computations, or real-time data processing systems.
Here’s an example of how easy it is to spin up goroutines and handle concurrency in Go:
package main
import (
"fmt"
"time"
)
func printMessage(msg string) {
for i := 0; i < 5; i++ {
fmt.Println(msg)
time.Sleep(time.Millisecond * 500) // Wait for 500 milliseconds
}
}
func main() {
go printMessage("Hello from goroutine!") // Launch a goroutine
printMessage("Hello from main thread!") // Execute in the main thread
// Allow the goroutine to finish before exiting the program
time.Sleep(time.Second * 3)
}
Example Breakdown
In this simple program, we launch a goroutine using the go keyword. This allows the printMessage function to run concurrently alongside the main function, making Go’s concurrency model incredibly easy to use.
There’s no complex setup, no dealing with threads, and no need to manually manage synchronization—just simple, clean code that works.
Built for Modern Software Development
Go also excels in building cloud-native applications, with first-class support for Docker and Kubernetes. Its fast compile times and cross-compilation features make it an ideal language for modern development workflows. This is one of the reasons I’ve started using Go for projects like building interactive dashboards and developing dev tools.
Conclusion
Go’s combination of simplicity, performance, and concurrency model is why I love using it. It allows me to build tools quickly and efficiently while handling complex tasks under the hood.
If you haven’t tried Go yet, I highly recommend giving it a shot—you might just fall in love with it like I did.